Electron AppSend Menu Command to Angular App
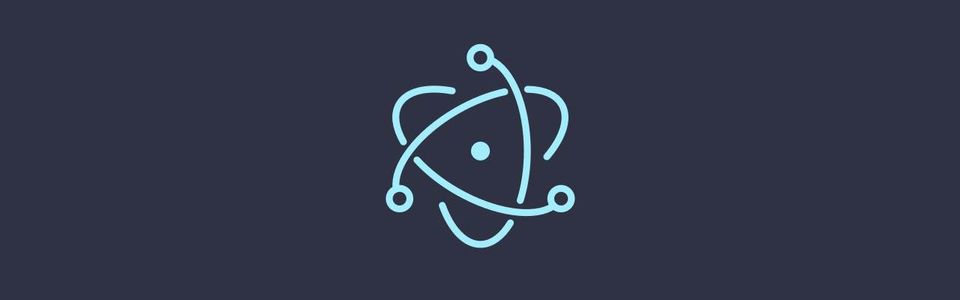
TL;DR: In the main process use mainWindow.webContents.send('cmd')
and in your Angular component (renderer process) bind it to a method with ipcRenderer.on('cmd', this.open.bind(this))
(where open()
is a method).
I need to make my angular app work when a menu item is clicked (think: I click on "Open" and my angular app needs to perform the actual operation). The problem I've encountered is the menu actions happen in the main process while my angular code lives in the renderer process. Thus, I need to make the two communicate :)
In my main code, I have something like:
fileMenu.submenu.find(item => item.label === 'Open').click = () =>
mainWindow.webContents.send('open-file')
which translates in:
Make it so when I click "Open" on my menu, I will send an IPC message containing "open-file" to my renderer process.
In my @Component
class constructor (renderer) I do the binding:
import { Component } from '@angular/core'
import { remote, ipcRenderer } from 'electron'
@Component({
// ...
})
export class AppComponent {
constructor(private dbService: DatabaseService) {
ipcRenderer.on('open-file', this.open.bind(this))
}
open() {
console.log('Open was triggered')
}
}
This practically says:
If I get an IPC message containing "open-file", call the
open()
method.
Now, you can receive commands from the menu too :) Just be careful to separate the concerns (e.g. you don't have access to app
in renderer ).
HTH,