Create a Background with Vertical Lines of Different Colours
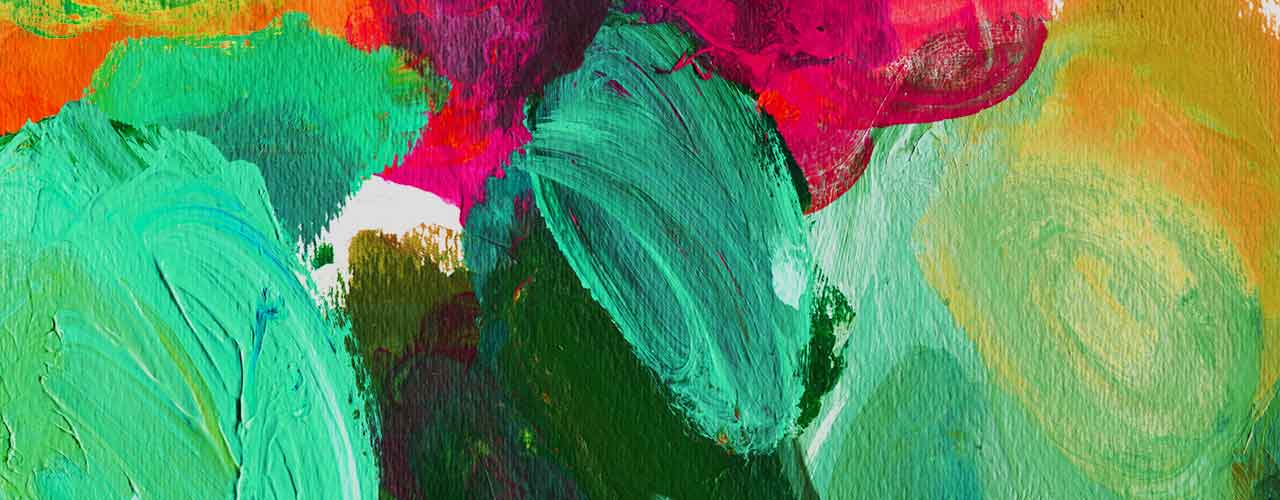
I've got a list of colours (RGB) in an array and I want to build a background with vertical lines, each representing a colour from my list.
The process is too simple to put in a TL;DR :)
If my list of colours is, Array<Vector3>
cols, then the process is as follows:
-
Create a pixmap:
Pixmap pix = new Pixmap(cols.size, 1, Pixmap.Format.RGB888);
-
Set one pixel with the corresponding colour in the list:
Vector3 color; for (int i = 0; i < cols.size; i++) { color = cols.get(i); pix.drawPixel(i, 0, Color.rgba8888(color.x, color.y, color.z, 1)); }
-
Create a texture off the pixmap:
Texture texture = new Texture(pix);
-
Create a Sprite and resize it:
Sprite sprite = new Sprite(texture); sprite.setSize(mApp.camera.viewportWidth, mApp.camera.viewportHeight); sprite.setPosition(0, 0);
This will create a sprite as large as your screen.
Note: You'll need to declare the sprite as a class member because you need to use it in render() .
-
In render() / draw() , render :) :
sprite.draw(batch);
Now, when you render, you'll get the striped background.
Horizontal stripes
If you want horizontal stripes, then all you need to do is to create a vertical pixmap:
Pixmap pix = new Pixmap(1, cols.size, Pixmap.Format.RGB888);
and fill it up accordingly (hint: invert the coordinates in drawPixel()
).
Note: The code above is taken from an Actor code.
HTH,